How to integrate your dApp with Biomapper on Filecoin
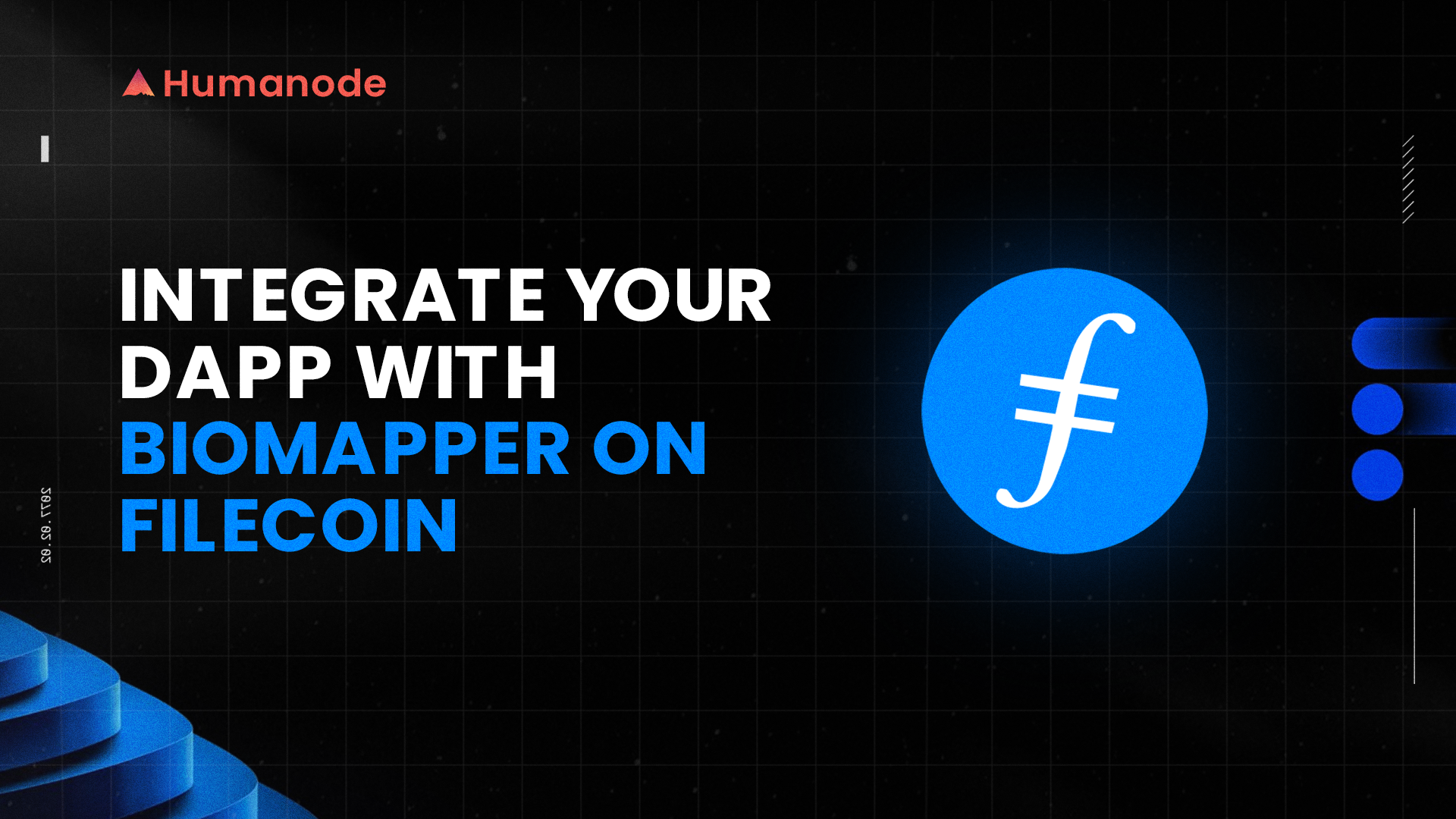
Filecoin is built for decentralized storage, letting users store and manage data without relying on centralized providers. It’s widely used by storage-focused dApps, DataDAOs, and compute-over-data applications that need reliable, distributed storage without a middleman calling the shots.
But here’s the problem: Sybil attacks. People can spin up multiple wallets to hoard free storage, manipulate governance, or game incentive programs. That’s a serious issue for dApps that need fair data access, transparent decision-making, and reliable resource distribution.
Humanode Biomapper helps fix this. It makes sure that each participating wallet belongs to a unique human – without KYC, identity documents, or personal data. Users stay private, dApps stay Sybil-resistant, and everyone gets a fair shot.
How dApps on Filecoin Benefit from Sybil Resistance
- Fair Storage Access → Prevents users from creating multiple accounts to unfairly take up free or subsidized storage.
- Secure Governance in DataDAOs → Enforces one-person-one-vote, stopping whales from manipulating decisions.
- Compute Resource Fairness → Ensures users providing or accessing compute-over-data networks are unique individuals, preventing abuse of reward incentives.
- Transparent Grant & Reward Distribution → Stops bots from gaming funding programs meant for real contributors.
For Filecoin developers, integrating Biomapper means a more secure, Sybil-resistant dApp ecosystem that protects incentives, governance, and storage fairness.
Getting Started
Before integrating Biomapper, get familiar with:
- Generations: Biomapper operates in fixed time-based generations. Each generation resets biometric mappings to maintain fresh Sybil resistance.
- Integration Flow: Users verify uniqueness once, bridge their biomapping to Filecoin, and can be verified across multiple dApps.
What You Need to Do
- Write a smart contract that interacts with the Bridged Biomapper on Filecoin.
- Add a link to the Biomapper UI on your frontend so users can complete their biomapping.
Development
Smart Contract Integration
Step 1: Install the Biomapper SDK
First, install the Biomapper SDK, which provides APIs for interacting with Humanode’s Bridged Biomapper on Filecoin.
Using npm:
npm install --save @biomapper-sdk/core @biomapper-sdk/libraries @biomapper-sdk/events
Using yarn:
yarn add @biomapper-sdk/core @biomapper-sdk/libraries @biomapper-sdk/events
Using Foundry:
forge install humanode-network/biomapper-sdk
Step 2: Import Biomapper Interfaces and Libraries
Inside your Solidity smart contract, import the necessary modules:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
import { IBridgedBiomapperRead } from "@biomapper-sdk/core/IBridgedBiomapperRead.sol";
import { IBiomapperLogRead } from "@biomapper-sdk/core/IBiomapperLogRead.sol";
import { BiomapperLogLib } from "@biomapper-sdk/libraries/BiomapperLogLib.sol";
These imports let your contract check user uniqueness and access biomapping logs.
Step 3: Connect Your Smart Contract to Bridged Biomapper
Since Bridged Biomapper is already deployed on Filecoin, you can interact with it directly – no need to deploy your own.
Example: Verifying Unique Users in a Decentralized Storage Service
pragma solidity ^0.8.0;
import "@biomapper-sdk/core/IBridgedBiomapperRead.sol";
contract StorageAccess {
IBridgedBiomapperRead public biomapper;
constructor(address _biomapperAddress) {
biomapper = IBridgedBiomapperRead(_biomapperAddress);
}
function isUniqueUser(address user) public view returns (bool) {
return biomapper.isBridgedUnique(user);
}
}
This contract:
- Connects to the Bridged Biomapper contract on Filecoin.
- Ensures only unique users can access subsidized or free storage.
Step 4: Using Mock Contracts for Local Development
For local testing, use the MockBridgedBiomapper contract to simulate biomapping verification before deploying to testnet/mainnet.
Example usage:
function generationsBridgingTxPointsListItem(uint256 ptr) external view returns (GenerationBridgingTxPoint memory);
Refer to the Biomapper SDK Docs for details on using mock contracts.
Frontend Integration
To complete the verification flow, add a link to the Biomapper UI so users can verify their uniqueness.
Example UI Implementation:
< a href="https://biomapper.hmnd.app" target="_blank">Verify Your Uniqueness< /a>
Once users verify their biomapping, they can return to your dApp and interact with your smart contract.
Deployment, Testing, and Rollout
Step 1: Deploy Your Smart Contract
Deploy your contract to Filecoin mainnet and connect it to Bridged Biomapper.
Step 2: Retrieve and Use Contract Addresses
Retrieve the latest contract addresses from the Biomapper SDK.
Step 3: Connect Frontend to Mainnet Biomapper
Ensure your frontend links users to the correct mainnet Biomapper UI before launching.
Step 4: Test Your Integration
- Use testnet environments to verify biomapping checks.
- Confirm that users can verify uniqueness and interact with smart contracts.
- Monitor transactions and logs to ensure proper execution.
Post-Rollout
- App Listing: Want your dApp featured in the Biomapper ecosystem? Contact the Humanode team.
- Ongoing Support: Keep your contract updated with the latest Biomapper SDK improvements for better security and functionality.
By integrating Biomapper, your dApp becomes Sybil-resistant while preserving user privacy. Whether you're building a decentralized storage service, a DataDAO, or a compute-over-data network, Biomapper ensures that only real humans participate.