How to verify real users in your Arbitrum dApp?
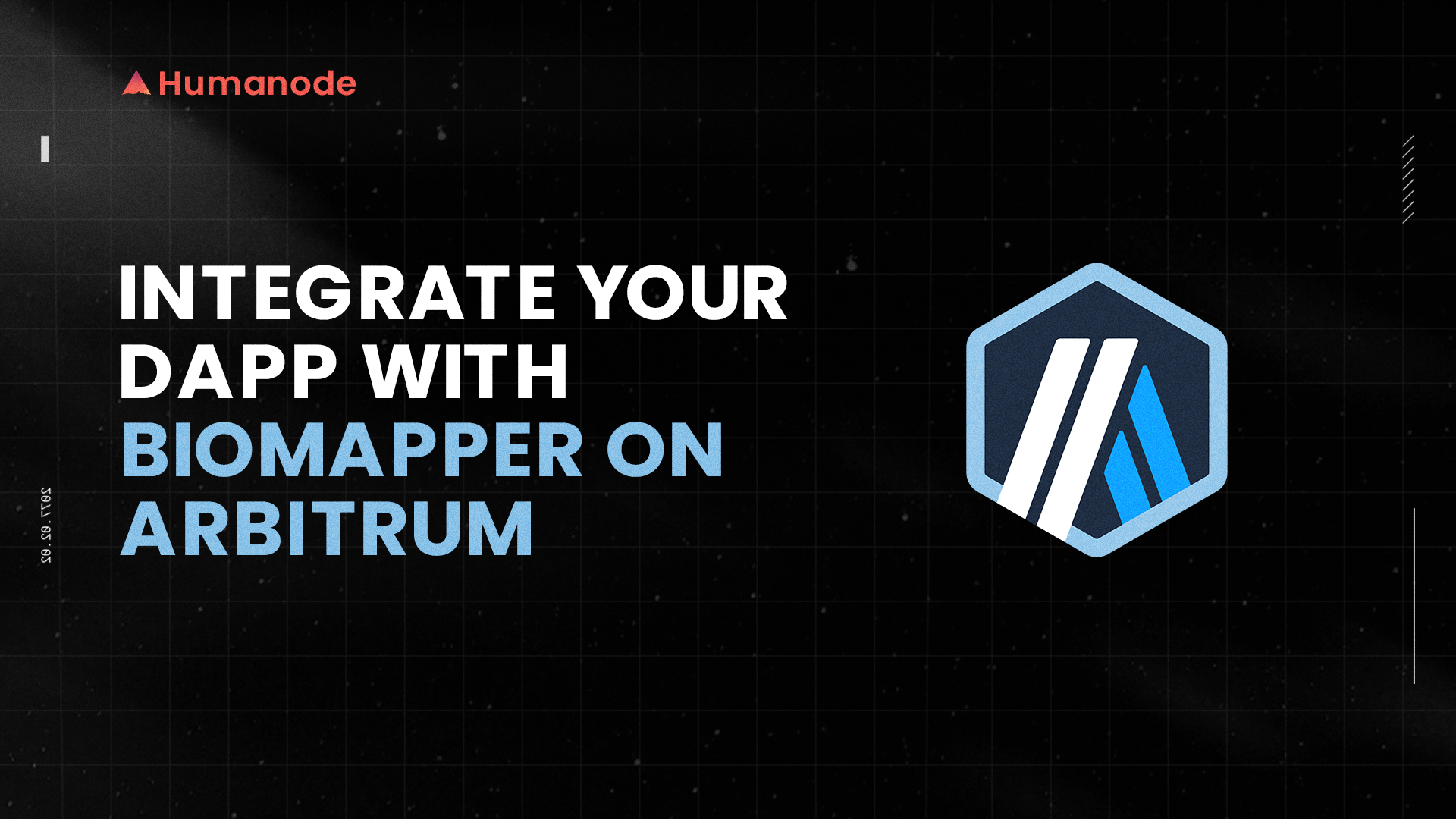
If you’re building on Arbitrum, you’re already familiar with faster transactions, cheaper gas fees, and the solid security of Ethereum. However, the openness and composability of Layer 2 also come with their own risks—specifically, Sybil attacks and difficulties ensuring user uniqueness without traditional identity checks.
Biomapper solves this problem by ensuring every wallet interacting with your dApp on Arbitrum is a unique human. There's no need for intrusive KYC or identity document verification—just a straightforward, privacy-preserving cryptographic proof of uniqueness.
Since Biomapper is already live on Arbitrum, integrating it into your dApp to verify unique participants is quick and straightforward. This guide will show you how.
Before integration, familiarize yourself with these Biomapper concepts:
- Generations: Biomapper verifies uniqueness within defined, time-based periods, regularly resetting biometric mappings.
- Integration Flow: Users verify once, bridge their biomapping to Arbitrum, and use it seamlessly across multiple dApps.
What to do:
- Write a smart contract that interacts with the Bridged Biomapper on HyperEVM.
- Add a link to the Biomapper UI on your front end so users can complete their biomapping.
Integration steps
Step 1: Install Biomapper SDK
Install Biomapper SDK in your project.
Using npm:
npm install --save @biomapper-sdk/core @biomapper-sdk/libraries @biomapper-sdk/events
Using yarn:
yarn add @biomapper-sdk/core @biomapper-sdk/libraries @biomapper-sdk/events
Using Foundry:
forge install humanode-network/biomapper-sdk
Step 2: Import required Interfaces
Include Biomapper interfaces in your smart contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
import { IBridgedBiomapperRead } from "@biomapper-sdk/core/IBridgedBiomapperRead.sol";
import { IBiomapperLogRead } from "@biomapper-sdk/core/IBiomapperLogRead.sol";
import { BiomapperLogLib } from "@biomapper-sdk/libraries/BiomapperLogLib.sol";
Step 3: Connect to BridgedBiomapper on Arbitrum
Reference the existing Biomapper smart contract already deployed on Arbitrum.
Example: Governance Voting Contract
pragma solidity ^0.8.0;
import "@biomapper-sdk/core/IBridgedBiomapperRead.sol";
contract GovernanceVoting {
IBridgedBiomapperRead public biomapper;
mapping(address => bool) public hasVoted;
constructor(address _biomapperAddress) {
biomapper = IBridgedBiomapperRead(_biomapperAddress);
}
function vote(uint proposalId) external {
require(biomapper.isBridgedUnique(msg.sender), "User not verified as unique");
require(!hasVoted[msg.sender], "User already voted");
hasVoted[msg.sender] = true;
// Implement voting logic here
}
}
This contract ensures voters are uniquely verified without requiring personal identity information.
Step 4: Local testing with mock contracts
Use the MockBridgedBiomapper for local development testing:
function generationsBridgingTxPointsListItem(uint256 ptr) external view returns (GenerationBridgingTxPoint memory);
Check Biomapper SDK documentation for more details.
Frontend integration
Allow users to verify uniqueness directly via your dApp:
< a href="https://biomapper.hmnd.app" target="_blank">Verify Your Uniqueness< / a>
Users return seamlessly to your dApp once verified.
Deployment and Rollout
- Deploy your contracts to Arbitrum.
- Connect to the correct Biomapper contract addresses.
- Update your frontend link to the Biomapper mainnet verification app.
- Thoroughly test integration on the Arbitrum testnet.
Post-deployment
- Consider listing your dApp within the Humanode Biomapper ecosystem.
- Regularly update according to Biomapper SDK releases.
With Biomapper integrated into your Arbitrum dApp, you can ensure authentic user interactions, robust Sybil resistance, and user privacy – all without intrusive KYC processes.