How to enable Sybil resistance in your dApps on Sonic?
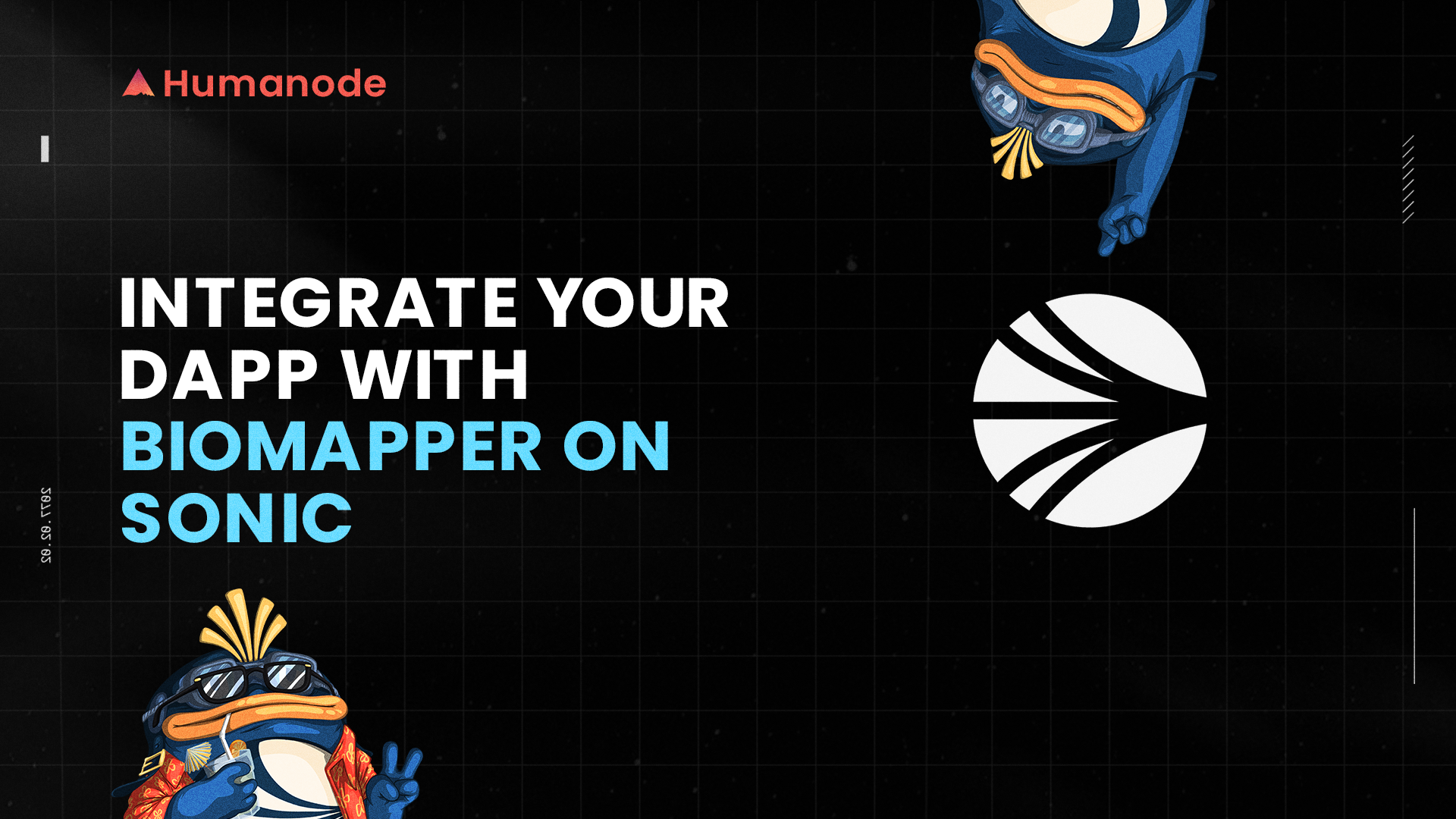
You're building on Sonic because you want high-speed transactions, instant finality, and Ethereum interoperability without sacrificing decentralization. However, with high throughput and low fees comes a challenge: Sybil attacks. When creating wallets is cheap, bad actors can spin up hundreds of accounts to exploit staking rewards, liquidity incentives, trading volume, and governance votes.
If you're running a DeFi protocol, governance system, or trading dApp, this is a serious problem. Multiple wallets controlled by the same entity can game rewards, manipulate governance decisions, and create fake trading activity, hurting real users and making your protocol less reliable.
That’s where Humanode Biomapper comes in. It lets you verify that each wallet belongs to a unique human without needing KYC, documents, or personal data. Just privacy-preserving cryptographic proof of uniqueness, ensuring fair participation without compromising user anonymity.
Why Sybil-resistance matters for dApps on Sonic
- Staking & Yield Farming: Stops multi-wallet farming of staking rewards.
- Governance Models: Enforces 1:1 human-to-vote mapping, eliminating governance manipulation.
- Decentralized Marketplaces: Ensures one verified trader per identity, stopping wash trading.
- High-Frequency Trading (HFT) Platforms: Protects against bots manipulating volume-based rewards.
- Cross-Chain Asset Transfers: Verifies real users, reducing the risk of fraudulent Sybil-based transactions.
How to enable Sybil-resistance in your dApps on Sonic
Before integrating Biomapper, familiarize yourself with core concepts such as:
- Generations: Biomapper operates in fixed time-based generations. Each generation resets biometric mappings.
- Integration Flow: Users verify uniqueness once, bridge their biomapping to Sonic, and can be verified across multiple dApps.
What You Need to Do
- Write a smart contract that interacts with the Bridged Biomapper on Base.
- Add a link to the Biomapper UI on your frontend so users can complete their biomapping.
Integration steps
1. Install the Biomapper SDK
The Biomapper SDK lets you verify user uniqueness through the BridgedBiomapper contract on Sonic.
Using npm:
npm install --save @biomapper-sdk/core @biomapper-sdk/libraries @biomapper-sdk/events
Using yarn:
yarn add @biomapper-sdk/core @biomapper-sdk/libraries @biomapper-sdk/events
Using Foundry:
forge install humanode-network/biomapper-sdk
2. Import Biomapper interfaces & libraries
In your Solidity contract, import the necessary interfaces:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
import { IBridgedBiomapperRead } from "@biomapper-sdk/core/IBridgedBiomapperRead.sol";
import { IBiomapperLogRead } from "@biomapper-sdk/core/IBiomapperLogRead.sol";
import { BiomapperLogLib } from "@biomapper-sdk/libraries/BiomapperLogLib.sol";
These imports expose read-access functions to check a wallet’s biomapping status and uniqueness.
3. Connect to BridgedBiomapper
Sonic already has Bridged Biomapper deployed, so you don’t need to deploy your own; just reference the existing contract.
Example: Staking Rewards Contract with Biomapper Verification
pragma solidity ^0.8.0;
import "@biomapper-sdk/core/IBridgedBiomapperRead.sol";
import "@openzeppelin/contracts/token/ERC20/IERC20.sol";
import "@openzeppelin/contracts/token/ERC20/utils/SafeERC20.sol";
contract SybilResistantStaking {
using SafeERC20 for IERC20;
IBridgedBiomapperRead public biomapper;
IERC20 public immutable STAKING_TOKEN;
mapping(address => uint256) public stakedAmount;
constructor(address _biomapperAddress, address _stakingToken) {
biomapper = IBridgedBiomapperRead(_biomapperAddress);
STAKING_TOKEN = IERC20(_stakingToken);
}
function stake(uint256 amount) external {
require(biomapper.isBridgedUnique(msg.sender), "User is not unique");
STAKING_TOKEN.safeTransferFrom(msg.sender, address(this), amount);
stakedAmount[msg.sender] += amount;
}
}
This contract ensures that only unique humans can stake tokens, stopping multi-wallet farming.
4. Local testing with mock contracts
For local testing, use the MockBridgedBiomapper contract to simulate biomapping without hitting mainnet.
Example:
function generationsBridgingTxPointsListItem(uint256 ptr) external view returns (GenerationBridgingTxPoint memory);
Refer to Biomapper SDK Docs for full details on using mocks.
Frontend integration
To complete user verification, link to the Biomapper App in your dApp UI.
Example Frontend Integration:
< a href="https://biomapper.hmnd.app" target="_blank">Verify Your Uniqueness< /a>
Once users complete their uniqueness verification, they return to your dApp and interact Sybil-free.
Deployment & rollout
1. Deploy the Smart contract
Deploy your staking/governance/trading contract to Sonic Mainnet and link it to BridgedBiomapper.
2. Retrieve the BridgedBiomapper contract address
Find the latest contract addresses from the Biomapper SDK.
3. Update frontend to Biomapper App
Ensure your UI links to the mainnet Biomapper App.
4. Test before deployment
- Use Sonic testnet for pre-launch validation.
- Confirm that biomapping checks prevent multi-wallet exploitation.
- Log interactions to monitor anomalies in biomapping verification.
Post-deployment & maintenance
- dApp Listing → Get featured in Humanode’s Biomapper ecosystem.
- Ongoing Updates → Stay aligned with Biomapper SDK improvements.
If you're building on Sonic, you're already prioritizing speed and efficiency. But for your dApp to be secure, fair, and Sybil-resistant, you need to ensure that each participant is a real, unique human.
Biomapper does exactly that: no KYC, no identity exposure, just pure cryptographic verification. Whether you’re building a staking platform, governance system, or DeFi protocol, this is a critical layer of security that protects your ecosystem from bots and multi-wallet exploiters.
Ready to integrate?
Get started here: